clojure2d.extra.glitch
Various glitching pixel filters or functions
Filter
Use following filters with filter-channels function.
- Slitscan - x/y slitscan simulation based on wave functions
- Shift-channels - just shift channels
- Mirror - mirror image along different axes
- Slitscan2 - slitscan simulation based on vector fields
- Fold - apply vector field on the image
- Pix2line - convert pixel into horizontal line
Machines
Short sketches operating on images/pixels.
- Blend - compose two images in glitchy way
All filters are equiped with random configuration generator.
Categories
Other vars: blend-machine blend-machine-random-config fold fold-random-config imgslicer mirror mirror-random-config mirror-types pix2line pix2line-random-config shift-channels shift-channels-random-config slitscan slitscan-random-config slitscan2 slitscan2-random-config
Code snippets
Save glitch
(defn save-result
[f params & opts]
(let [n (str "images/glitch/" (first opts) ".jpg")]
(save f (str "docs/" n))
(str "../" n)))
blend-machine
(blend-machine p1 p2)
(blend-machine {:keys [switch? in1-cs in2-cs out-cs in1-to? in2-to? out-to? blend-ch1 blend-ch2 blend-ch3]} p1 p2)
Blend two Pixels
based on configuration.
The idea is to compose channels separately in different color spaces.
Full flow does following steps:
- convert inputs to (or from) selected color spaces
- compose each channel separately using different method
- convert output to (or from) selected color space
Parametrization:
:switch?
- reverse input order:in1-cs
- color space for first image:in2-cs
- color space for second image:out-cs
- color space for output:in1-to?
,:in2-to?
,:cs-to?
- which conversion to select: to color space or from color space:blend-ch1
,:blend-ch2
,:blend-ch3
- blend methods for each channel
Examples
Usage
(save-result (let [blend-conf {:blend-ch3 :hardmix,
:out-cs :Cubehelix,
:blend-ch2 :mlinearburn,
:switch? true,
:out-to? true,
:in1-cs :LUV,
:in1-to? true,
:in2-cs :XYZ,
:blend-ch1 :mdodge,
:in2-to? true}
p2l-conf {:nx 9,
:ny 3,
:scale 4.2,
:tolerance 37,
:nseed -1,
:whole true,
:shiftx 0.66,
:shifty 0.46}
p2 (p/filter-channels (pix2line p2l-conf) samurai)]
(blend-machine blend-conf samurai p2))
...)
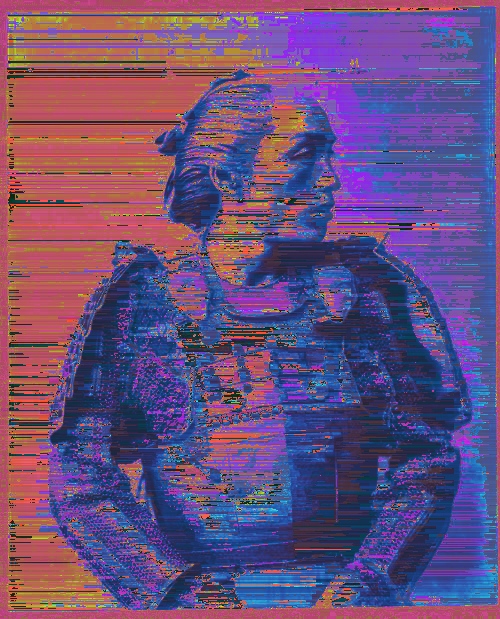
blend-machine-random-config
(blend-machine-random-config)
Random configuration for blend machine.
Examples
Usage
(blend-machine-random-config)
;;=> {:blend-ch1 nil,
;;=> :blend-ch2 :difference,
;;=> :blend-ch3 nil,
;;=> :in1-cs :RYB,
;;=> :in1-to? true,
;;=> :in2-cs nil,
;;=> :in2-to? true,
;;=> :out-cs :RYB,
;;=> :out-to? false,
;;=> :switch? false}
fold
(fold {:keys [fields r]})
(fold)
Folding filter based on vector fields.
Parameters:
:fields
- vector fields configuration combine:r
- range value 1.0-3.0
Examples
Fold with random config
(save-result (p/filter-channels (fold) samurai) ...)
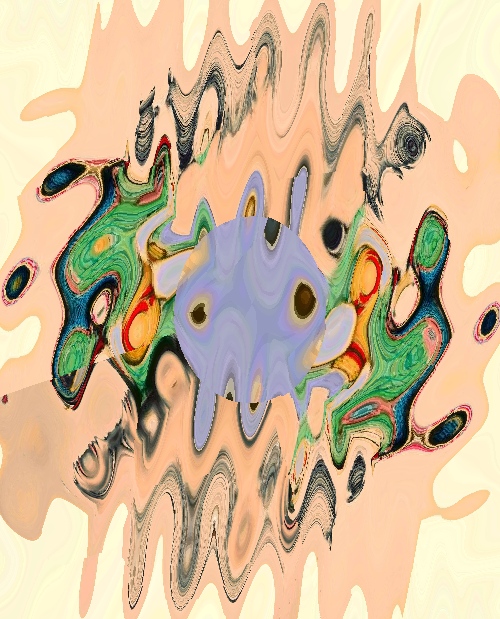
Provided config
(save-result
(let [conf
{:fields
{:type :operation,
:name :comp,
:var1 {:type :variation,
:name :pressure-wave,
:amount 1.0,
:config {:x-freq -0.27, :y-freq 5.92}},
:var2
{:type :variation, :name :besselj, :amount 1.0, :config {}},
:amount 1.0},
:r 4.0}]
(p/filter-channels (fold conf) samurai))
...)
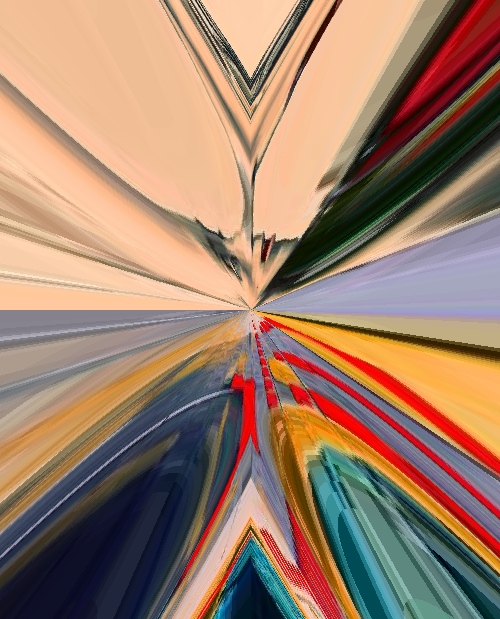
Provided config with different range value for each channel
(save-result
(let [conf
{:fields {:type :operation,
:name :comp,
:var1 {:type :variation,
:name :perlin2,
:amount 1.0,
:config {:seed 112, :scale -1.13, :octaves 4}},
:var2 {:type :variation,
:name :exponential,
:amount 1.0,
:config {}},
:amount 1.0},
:r 2.0}]
(p/filter-channels (fold (assoc conf :r 1.9))
(fold (assoc conf :r 2.0))
(fold (assoc conf :r 2.1))
nil
samurai))
...)
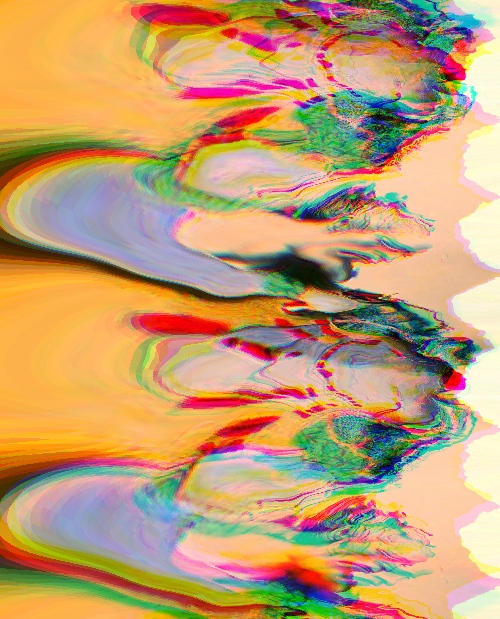
fold-random-config
Generate random configuration for vector fields slitscan.
r
- field range (default 2.0)d
- fields configuration depth (default up to 3)
Examples
Usage
(fold-random-config)
;;=> {:fields {:amount 1.0,
;;=> :name :deriv,
;;=> :step 0.7413797709592066,
;;=> :type :operation,
;;=> :var {:amount 0.922636679651495,
;;=> :name :add,
;;=> :type :operation,
;;=> :var1 {:amount -0.1980211450305598,
;;=> :config {},
;;=> :name :gridout,
;;=> :type :variation},
;;=> :var2 {:amount 0.8858291094138302,
;;=> :config {},
;;=> :name :cross,
;;=> :type :variation}}},
;;=> :r 2.0}
(fold-random-config 3.1234)
;;=> {:fields
;;=> {:amount 0.43443906817713573,
;;=> :name :add,
;;=> :type :operation,
;;=> :var1 {:amount -0.9988505611206575,
;;=> :name :comp,
;;=> :type :operation,
;;=> :var1
;;=> {:amount 1.0,
;;=> :name :comp,
;;=> :type :operation,
;;=> :var1
;;=> {:amount 1.0, :config {}, :name :swirl, :type :variation},
;;=> :var2 {:amount 1.0,
;;=> :config {:scale-r-left 1.3442689559877834,
;;=> :scale-r-right 1.3442689559877834,
;;=> :scale-x -1.135631932161936,
;;=> :shift-t 0.726975699980737},
;;=> :name :heartwf,
;;=> :type :variation}},
;;=> :var2 {:amount 1.0,
;;=> :config {:a 1.2089700605456892,
;;=> :b 0.7535182079698477,
;;=> :c -0.8550332281801727,
;;=> :d 0.20858091601997275},
;;=> :name :clifford,
;;=> :type :variation}},
;;=> :var2 {:amount 1.3029682053082072,
;;=> :config {},
;;=> :name :rays3,
;;=> :type :variation}},
;;=> :r 3.1234}
(fold-random-config 3.1234 0)
;;=> {:fields {:amount 1.0,
;;=> :config {:inverse true,
;;=> :p1 -2.5436391813388575,
;;=> :p2 1.6369101550213472},
;;=> :name :joukowski,
;;=> :type :variation},
;;=> :r 3.1234}
imgslicer
(imgslicer pixels {:keys [distance threshold min-size mode], :or {distance v/dist, threshold 200, min-size 500, mode :color}, :as options})
mirror
(mirror t)
(mirror)
Mirror image for given (or random) mirroring functions.
Examples
Random mirror
(save-result (p/filter-channels (mirror) samurai) ...)
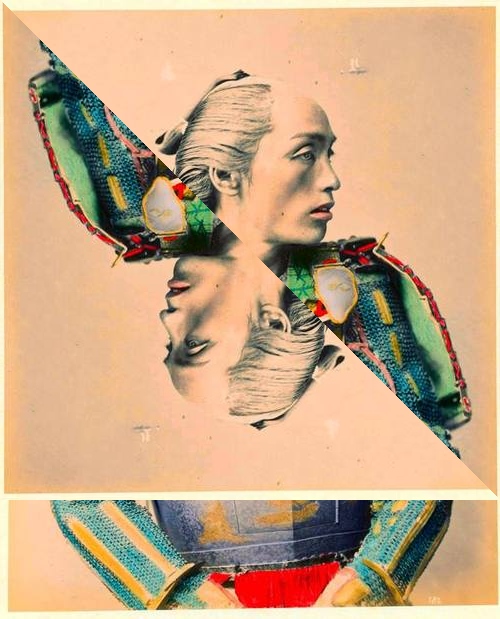
Mirror R(ight) to left
(save-result (p/filter-channels (mirror :R) samurai) ...)
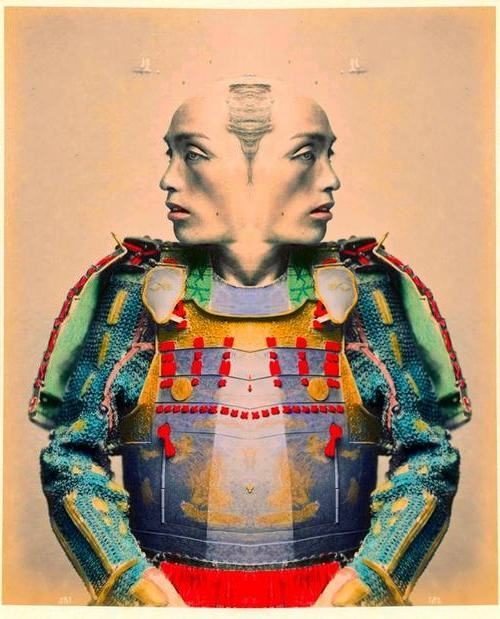
Mirror each channel separately
(save-result
(p/filter-channels (mirror :L) (mirror :R) (mirror :U) nil samurai)
...)
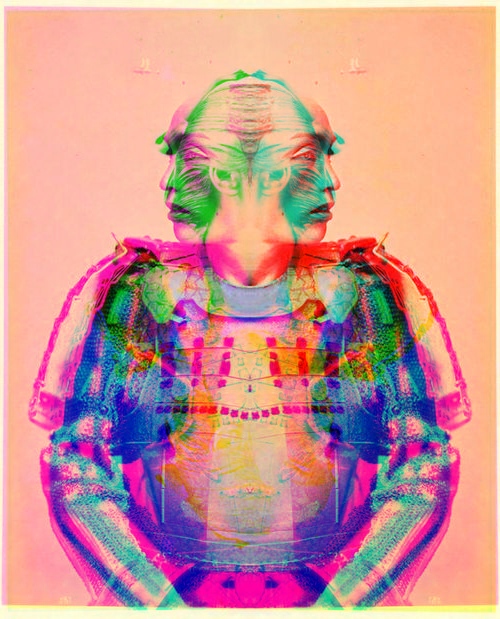
mirror-random-config
(mirror-random-config)
Generate random mirroring functions.
Examples
Usage
(mirror-random-config)
;;=> :UR
(mirror-random-config)
;;=> :U
(mirror-random-config)
;;=> :RDR
mirror-types
Map of names and mirroring functions
Examples
List of mirror function keys
(sort (keys mirror-types))
;;=> (:D :DL
;;=> :DL2 :DR
;;=> :DR2 :L
;;=> :R :RDL
;;=> :RDR :RUL
;;=> :RUR :SDL
;;=> :SDL2 :SDR
;;=> :SDR2 :SUL
;;=> :SUL2 :SUR
;;=> :SUR2 :U
;;=> :UL :UL2
;;=> :UR :UR2)
pix2line
(pix2line)
(pix2line {:keys [tolerance whole], :as config})
Pix2line effect. Convert pixels to lines.
Parametrization:
:nx
,:ny
- grid size:scale
- grid scaling factor:tolerance
- factor which regulates when start new line:nseed
- noise seed:whole
- skip lines or not:shiftx
,:shifty
- noise shift
Examples
Random pix2line
(save-result (p/filter-channels (pix2line) samurai) ...)
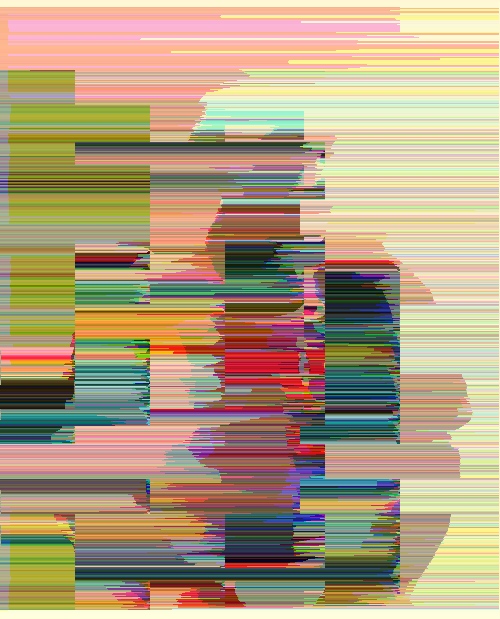
With configuration, slightly modified for each channel
(save-result
(let [conf {:nx 9,
:ny 3,
:scale 4.2,
:tolerance 37,
:nseed -1,
:whole true,
:shiftx 0.66,
:shifty 0.46}]
(p/filter-channels (pix2line conf)
(pix2line (assoc conf :scale 1))
(pix2line (assoc conf :scale 10 :tolerance 100))
nil
samurai))
...)
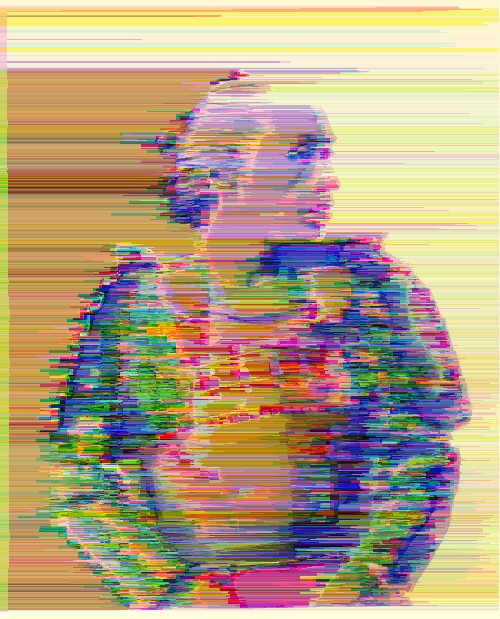
pix2line-random-config
(pix2line-random-config)
Make random config for pix2line.
Examples
Usage
(pix2line-random-config)
;;=> {:nseed -1654163163,
;;=> :nx 51,
;;=> :ny 32,
;;=> :scale 4.485852842368873,
;;=> :shiftx 0.09824219470759632,
;;=> :shifty 0.2327535881966133,
;;=> :tolerance 42,
;;=> :whole true}
shift-channels
(shift-channels)
(shift-channels {:keys [x-shift y-shift], :or {x-shift 0.05, y-shift -0.05}})
Shift channels by given amount.
Parameters:
:x-shift
- shift amount along x axis:y-shift
- shift amount along y axis
Examples
Shift red and blue channels
(save-result
(p/filter-channels (shift-channels) nil (shift-channels) nil samurai)
...)
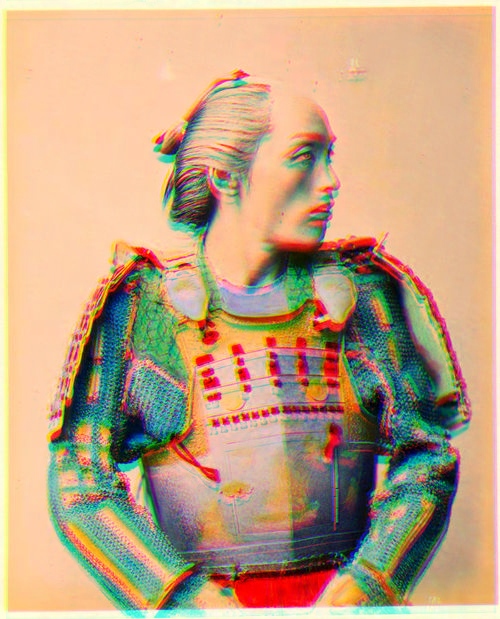
Shift hue and saturation channels
(save-result (let [filt (shift-channels {:x-shift -0.2, :y-shift 1.0})]
(->> samurai
(p/filter-colors c/to-HSV*)
(p/filter-channels filt filt nil nil)
(p/filter-colors c/from-HSV*)))
...)
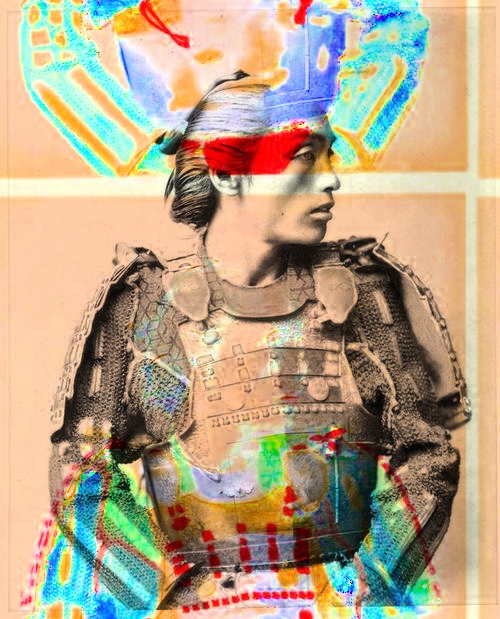
shift-channels-random-config
(shift-channels-random-config)
(shift-channels-random-config spread)
Random shift values along x and y axes.
Optionally provide spread
parameter to define maximum shift value.
Examples
Usage
(shift-channels-random-config)
;;=> {:x-shift -0.07336990662134679, :y-shift 0.04544682398873695}
(shift-channels-random-config 100)
;;=> {:x-shift -7.422686013277428, :y-shift 74.49102177395997}
slitscan
(slitscan)
(slitscan {:keys [x y], :or {x [(make-random-wave)], y [(make-random-wave)]}})
Create slitscan filter funtion.
Config is a map each axis has it’s own list of maps defining waves. Each map contains:
:wave
- oscillator name (see oscillators.:freq
- wave frequency:amp
- wave amplitude:phase
- wave phase (0-1).
Examples
Random slitscan
(save-result (p/filter-channels (slitscan) samurai) ...)
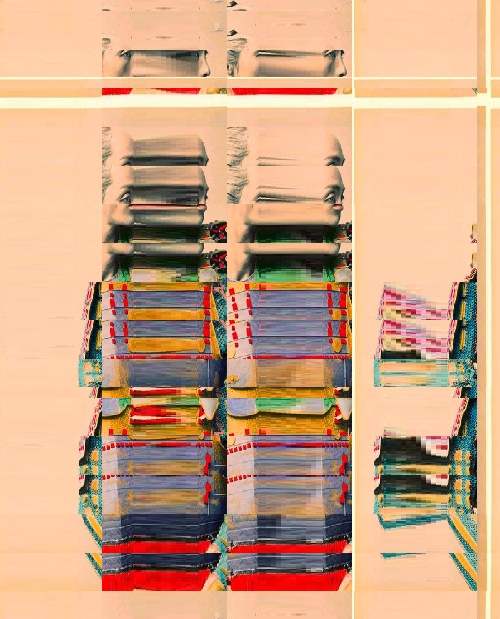
Slitscan with parameters
(save-result
(p/filter-channels
(slitscan {:x [{:wave :triangle, :freq 4, :amp 0.25, :phase 0.8}],
:y [{:wave :sin, :freq 2, :amp 0.5, :phase 0.5}]})
samurai)
...)
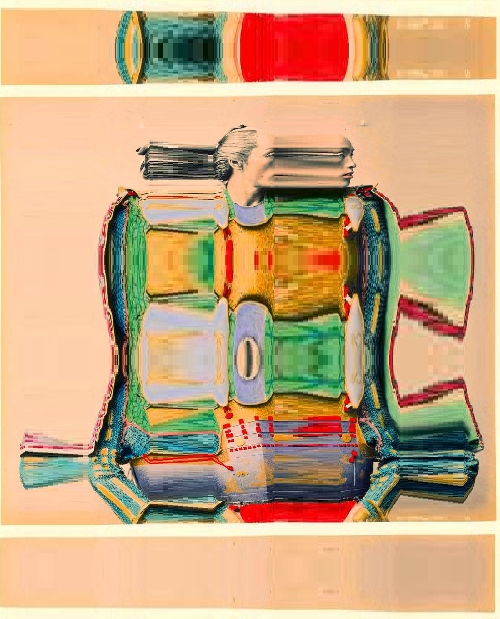
slitscan-random-config
(slitscan-random-config nx ny)
(slitscan-random-config)
Create list of random waves for each axis separately.
Optionally you can pass number of waves to create for each axis.
Examples
Usage
(slitscan-random-config)
;;=> {:x ({:amp 1.0, :freq 1, :phase 0.9241246438006804, :wave :constant}
;;=> {:amp 0.5, :freq 2, :phase 0.5759937073440069, :wave :square}),
;;=> :y
;;=> ({:amp 0.25, :freq 4, :phase 0.7224701755541347, :wave :cut-triangle}
;;=> {:amp 0.125, :freq 8, :phase 0.3633170590483046, :wave :noise})}
(slitscan-random-config 1 1)
;;=> {:x ({:amp 1.0, :freq 1, :phase 0.4257209942818533, :wave :noise}),
;;=> :y ({:amp 0.5, :freq 2, :phase 0.40037510860088343, :wave :triangle})}
slitscan2
(slitscan2 {:keys [fields r], :or {r 2.0, fields (var/random-configuration 0)}})
(slitscan2)
Slitscan filter based on vector fields.
Parameters:
:fields
- vector fields configuration combine:r
- range value 1.0-3.0
Examples
Slitscan with random config
(save-result (p/filter-channels (slitscan2) samurai) ...)
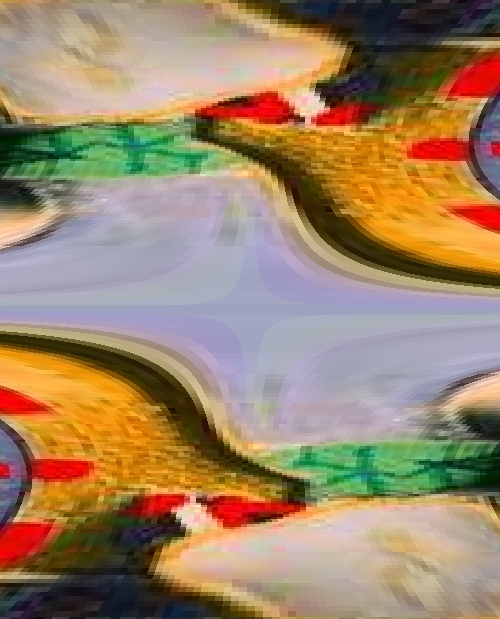
Provided config
(save-result
(let [conf
{:fields
{:type :operation,
:name :comp,
:var1 {:type :variation,
:name :pressure-wave,
:amount 1.0,
:config {:x-freq -0.27, :y-freq 5.92}},
:var2
{:type :variation, :name :besselj, :amount 1.0, :config {}},
:amount 1.0},
:r 4.0}]
(p/filter-channels (slitscan2 conf) samurai))
...)
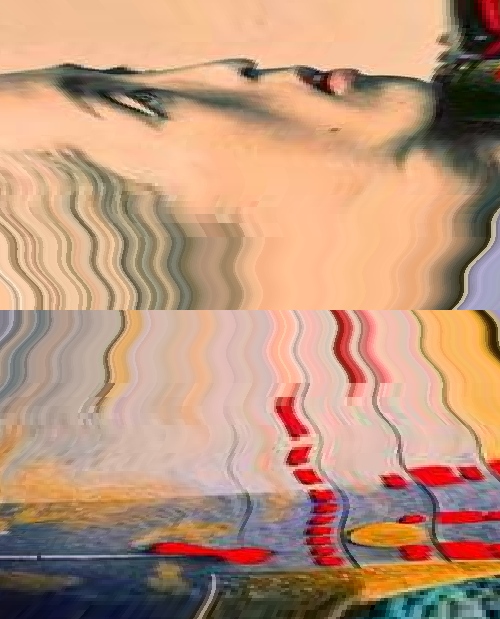
Provided config with different range value for each channel
(save-result
(let [conf
{:fields {:type :operation,
:name :comp,
:var1 {:type :variation,
:name :perlin2,
:amount 1.0,
:config {:seed 112, :scale -1.13, :octaves 4}},
:var2 {:type :variation,
:name :exponential,
:amount 1.0,
:config {}},
:amount 1.0},
:r 2.0}]
(p/filter-channels (slitscan2 (assoc conf :r 1.9))
(slitscan2 (assoc conf :r 2.0))
(slitscan2 (assoc conf :r 2.1))
nil
samurai))
...)
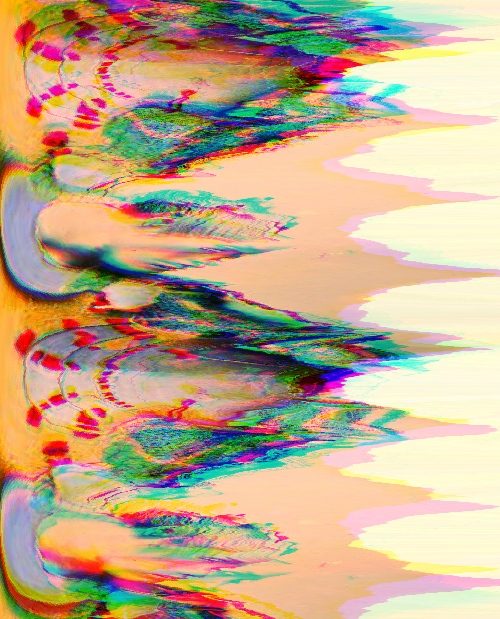
slitscan2-random-config
(slitscan2-random-config)
(slitscan2-random-config r)
(slitscan2-random-config r d)
Generate random configuration for vector fields slitscan.
r
- field range (default 2.0)d
- fields configuration depth (default up to 3)
Examples
Usage
(slitscan2-random-config)
;;=> {:fields {:amount 1.0,
;;=> :name :comp,
;;=> :type :operation,
;;=> :var1 {:amount 1.0,
;;=> :name :angles,
;;=> :type :operation,
;;=> :var1 {:amount 1.0,
;;=> :name :deriv,
;;=> :step 0.4866943903883673,
;;=> :type :operation,
;;=> :var {:amount 1.0,
;;=> :config {},
;;=> :name :flipy,
;;=> :type :variation}},
;;=> :var2 {:amount 1.0,
;;=> :config {:a 0.5519446526060249,
;;=> :b 0.6157800090173996,
;;=> :c -1.4234683216570923,
;;=> :d -0.15220974351257732,
;;=> :e 1.4482249308192476,
;;=> :f 1.6453981839047063,
;;=> :g 1.8409251785054788,
;;=> :h 1.231601984451795},
;;=> :name :disc3,
;;=> :type :variation}},
;;=> :var2 {:amount 1.0,
;;=> :config {:x1 0.8326870840061318,
;;=> :x2 0.6261931654789625,
;;=> :y1 0.17742712189118082,
;;=> :y2 1.5187363735816775},
;;=> :name :tanh2bs,
;;=> :type :variation}},
;;=> :r 2.0}
(slitscan2-random-config 3.1234)
;;=> {:fields {:amount 1.0,
;;=> :name :deriv,
;;=> :step 0.005327425261431854,
;;=> :type :operation,
;;=> :var {:amount 1.0,
;;=> :name :deriv,
;;=> :step 0.30569932787237186,
;;=> :type :operation,
;;=> :var {:amount 1.0,
;;=> :config {:gain 0.5978253690827111,
;;=> :generator :ridgemulti,
;;=> :interpolation :linear,
;;=> :lacunarity 1.731097743564447,
;;=> :noise-type :simplex,
;;=> :normalize? true,
;;=> :octaves 3,
;;=> :scale 0.6584231694752507,
;;=> :seed -404957211,
;;=> :warp-depth 1,
;;=> :warp-scale 0.0},
;;=> :name :general-noise2,
;;=> :type :variation}}},
;;=> :r 3.1234}
(slitscan2-random-config 3.1234 0)
;;=> {:fields {:amount 1.0,
;;=> :config {:k -0.5647225525175117},
;;=> :name :jacsn,
;;=> :type :variation},
;;=> :r 3.1234}